In the two weeks since the last update I have managed to get some of the sketch to run on a Circuit Playground Express and have that sketch report the led counter variable to the Processing sketch via serial communication.
The processing sketch (below) is still a bit crude as is the sketch running on the board – it visually displays the progress of each chunk of time by advancing a sphere from “Work” on the left to “Break” on the right of a graduated horizontal screen. I made this small and horizontal so it could take little real estate and be just above the task bar for quick checking.
The sketch running on the Circuit Playground still uses delays to control timing and this is holding back use of the two buttons on the printed housing. I was able to get the milli timer working on the Arduino Uno board and have that report to the processing sketch, but I needed the form factor of the CircuitPlayground with integrated neopixels for its compact size and fit into a small egg-shaped housing.
Currently the timer is 3 seconds per LED for the benefit of my impatient testing, but I’m updating it to a more respectable 5 min per LED which will be 2 Pomodoros. The flip action is handled by the Circuit Playground onboard IMU and senses the turnover to restart the timer – this does not interrupt the timer unfortunately, and revised sketch on the CP is needed to allow for this and the buttons to be able to interrupt the ongoing timer.
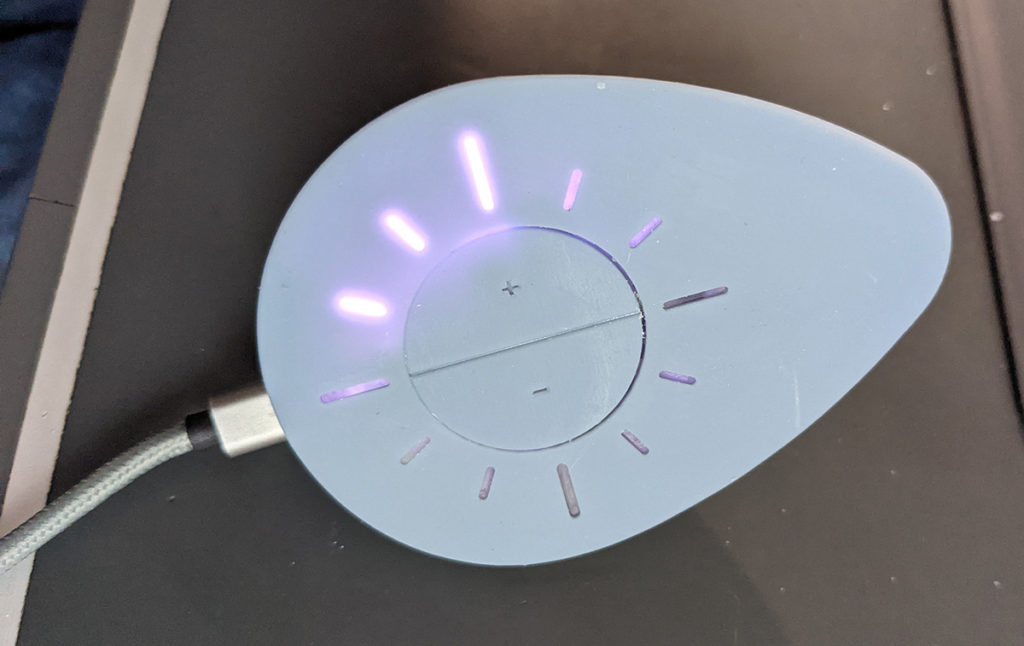
import processing.serial.*;
Serial arduinoConnection;
int sensor = 0;
int Y_AXIS = 1;
int X_AXIS = 2;
color b1, b2, c1, c2;
void setup() {
println( arduinoConnection.list() );
println(sensor);
size( 800, 200 );
textFont(createFont("Tahoma", 36));
arduinoConnection = new Serial(this, Serial.list()[1], 9600);
// Define colors
b1 = color(255);
b2 = color(100);
c1 = color(90, 102, 0);
c2 = color(0, 102, 153);
}
void draw() {
// Background
setGradient(0, 0, width/2, height, b1, b2, X_AXIS);
setGradient(width/2, 0, width/2, height, b2, b1, X_AXIS);
// Foreground
setGradient(20, 20, 740, 50, c1, c2, X_AXIS);
setGradient(20, 60, 740, 80, b1, b2, Y_AXIS);
textSize(20);
fill( 255 );
text("WORK", 50, 50);
text("BREAK",670,50);
float x = map( sensor, 0, 10, 0, width ); // change to fit your sensor values
float b = map( sensor, 0, 10, 0, 255 );
fill( 255-b, 0, b );
circle( x, height/2, 50 );
}
void serialEvent(Serial port) {
try {
String data = port.readStringUntil('\n');
if ( data != null ) {
data = trim(data);
if ( data.isEmpty() == false ) {
//println(data);
sensor = Integer.parseInt(data);
}
}
} catch(Exception ex) {
// handle the error
println(ex);
}
}
void setGradient(int x, int y, float w, float h, color c1, color c2, int axis ) {
noFill();
if (axis == Y_AXIS) { // Top to bottom gradient
for (int i = y; i <= y+h; i++) {
float inter = map(i, y, y+h, 0, 1);
color c = lerpColor(c1, c2, inter);
stroke(c);
line(x, i, x+w, i);
}
}
else if (axis == X_AXIS) { // Left to right gradient
for (int i = x; i <= x+w; i++) {
float inter = map(i, x, x+w, 0, 1);
color c = lerpColor(c1, c2, inter);
stroke(c);
line(i, y, i, y+h);
}
}
for(int n = 20; n < width; n += 40) {
// If 'i' divides by 20 with no remainder draw
// the first line, else draw the second line
if((n % 20) == 0) {
stroke(255);
line(n, 120, n, height/2);
} else {
stroke(153);
line(n, 90, n, 180);
}
}
}